Gemini API תומך ביצירת תמונות באמצעות Gemini 2.0 Flash Experimental וגם באמצעות Imagen 3. המדריך הזה יעזור לכם להתחיל לעבוד עם שני המודלים.
יצירת תמונות באמצעות Gemini
מודל Gemini 2.0 Flash Experimental תומך בפלט של טקסט ותמונות בתוך הטקסט. כך תוכלו להשתמש ב-Gemini כדי לערוך תמונות בשיחה או ליצור תוכן עם טקסט שמשולב בתמונות (לדוגמה, ליצור פוסט בבלוג עם טקסט ותמונות בפנייה אחת). כל התמונות שנוצרו כוללות סימן מים של SynthID, וגם תמונות ב-Google AI Studio כוללות סימן מים גלוי.
בדוגמה הבאה מוסבר איך להשתמש ב-Gemini 2.0 כדי ליצור פלט של טקסט ותמונה:
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
import base64
client = genai.Client()
contents = ('Hi, can you create a 3d rendered image of a pig '
'with wings and a top hat flying over a happy '
'futuristic scifi city with lots of greenery?')
response = client.models.generate_content(
model="gemini-2.0-flash-exp-image-generation",
contents=contents,
config=types.GenerateContentConfig(
response_modalities=['Text', 'Image']
)
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
image = Image.open(BytesIO((part.inline_data.data)))
image.save('gemini-native-image.png')
image.show()
const { GoogleGenerativeAI } = require("@google/generative-ai");
const fs = require("fs");
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY);
async function generateImage() {
const contents = "Hi, can you create a 3d rendered image of a pig " +
"with wings and a top hat flying over a happy " +
"futuristic scifi city with lots of greenery?";
// Set responseModalities to include "Image" so the model can generate an image
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp-image-generation",
generationConfig: {
responseModalities: ['Text', 'Image']
},
});
try {
const response = await model.generateContent(contents);
for (const part of response.response.candidates[0].content.parts) {
// Based on the part type, either show the text or save the image
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
const imageData = part.inlineData.data;
const buffer = Buffer.from(imageData, 'base64');
fs.writeFileSync('gemini-native-image.png', buffer);
console.log('Image saved as gemini-native-image.png');
}
}
} catch (error) {
console.error("Error generating content:", error);
}
}
generateImage();
curl -s -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash-exp-image-generation:generateContent?key=$GEMINI_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"contents": [{
"parts": [
{"text": "Hi, can you create a 3d rendered image of a pig with wings and a top hat flying over a happy futuristic scifi city with lots of greenery?"}
]
}],
"generationConfig":{"responseModalities":["Text","Image"]}
}' \
| grep -o '"data": "[^"]*"' \
| cut -d'"' -f4 \
| base64 --decode > gemini-native-image.png
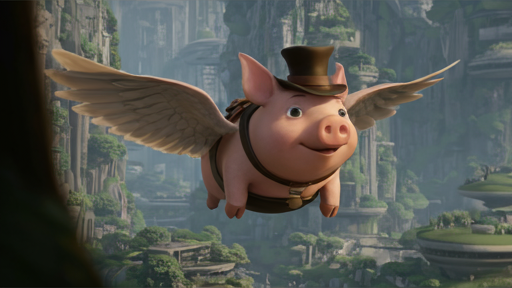
בהתאם להנחיה ולהקשר, Gemini תיצור תוכן במצבים שונים (טקסט לתמונה, טקסט לתמונה וטקסט וכו'). הנה כמה דוגמאות:
- טקסט לתמונה
- דוגמה להנחיה: "יצירת תמונה של מגדל אייפל עם זיקוקים ברקע".
- טקסט לתמונות וטקסט (מקובצים)
- הנחיה לדוגמה: "יצירת מתכון מאויר לפאייה".
- תמונות וטקסט לתמונות וטקסט (מקובצים)
- הנחיה לדוגמה: (עם תמונה של חדר מצויד) "איזה ספות בצבעים אחרים יתאימו למרחב שלי? יש לך אפשרות לעדכן את התמונה?"
- עריכת תמונות (טקסט ותמונה לתמונה)
- הנחיה לדוגמה: "עריכת התמונה הזו כך שתראה כמו קריקטורה"
- דוגמה להנחיה: [image of a cat] + [image of a pillow] + "Create a cross stitch of my cat on this pillow".
- עריכת תמונות במספר שלבים (צ'אט)
- הנחיות לדוגמה: [upload an image of a blue car.] "Turn this car into a convertible" "עכשיו משנים את הצבע לצהוב".
עריכת תמונות באמצעות Gemini
כדי לערוך תמונה, מוסיפים תמונה כקלט. בדוגמה הבאה מוסבר איך מעלים תמונות בקידוד base64. כדי לשלוח כמה תמונות ועומסי נתונים גדולים יותר, אפשר לעיין בקטע הזנת תמונה.
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
import PIL.Image
image = PIL.Image.open('/path/to/image.png')
client = genai.Client()
text_input = ('Hi, This is a picture of me.'
'Can you add a llama next to me?',)
response = client.models.generate_content(
model="gemini-2.0-flash-exp-image-generation",
contents=[text_input, image],
config=types.GenerateContentConfig(
response_modalities=['Text', 'Image']
)
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
image = Image.open(BytesIO(part.inline_data.data))
image.show()
const { GoogleGenerativeAI } = require("@google/generative-ai");
const fs = require("fs");
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY);
async function generateImage() {
// Load the image from the local file system
const imagePath = '/path/to/image.png';
const imageData = fs.readFileSync(imagePath);
const base64Image = imageData.toString('base64');
// Prepare the content parts
const contents = [
{ text: "Hi, This is a picture of me. Can you add a llama next to me?" },
{
inlineData: {
mimeType: 'image/png',
data: base64Image
}
}
];
// Set responseModalities to include "Image" so the model can generate an image
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp-image-generation",
generationConfig: {
responseModalities: ['Text', 'Image']
},
});
try {
const response = await model.generateContent(contents);
for (const part of response.response.candidates[0].content.parts) {
// Based on the part type, either show the text or save the image
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
const imageData = part.inlineData.data;
const buffer = Buffer.from(imageData, 'base64');
fs.writeFileSync('gemini-native-image.png', buffer);
console.log('Image saved as gemini-native-image.png');
}
}
} catch (error) {
console.error("Error generating content:", error);
}
}
generateImage();
IMG_PATH=/path/to/your/image1.jpeg
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
IMG_BASE64=$(base64 "$B64FLAGS" "$IMG_PATH" 2>&1)
curl -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash-exp-image-generation:generateContent?key=$GEMINI_API_KEY" \
-H 'Content-Type: application/json' \
-d "{
\"contents\": [{
\"parts\":[
{\"text\": \"'Hi, This is a picture of me. Can you add a llama next to me\"},
{
\"inline_data\": {
\"mime_type\":\"image/jpeg\",
\"data\": \"$IMG_BASE64\"
}
}
]
}],
\"generationConfig\": {\"responseModalities\": [\"Text\", \"Image\"]}
}" \
| grep -o '"data": "[^"]*"' \
| cut -d'"' -f4 \
| base64 --decode > gemini-edited-image.png
מגבלות
- כדי להשיג את הביצועים הטובים ביותר, מומלץ להשתמש בשפות הבאות: EN, es-MX, ja-JP, zh-CN, hi-IN.
- יצירת תמונות לא תומכת בקלט של אודיו או וידאו.
- יכול להיות שיצירת התמונות לא תמיד תגרום לפעולות הבאות:
- המודל יכול להפיק פלט של טקסט בלבד. נסו לבקש פלט של תמונות באופן מפורש (למשל, 'יצירת תמונה', 'הוספת תמונות תוך כדי עבודה', 'עדכון התמונה').
- יכול להיות שהמודל יפסיק ליצור תמונות באמצע התהליך. אפשר לנסות שוב או לנסות הנחיה אחרת.
- כשאתם יוצרים טקסט לתמונה, הכי טוב ליצור קודם את הטקסט ואז לבקש תמונה עם הטקסט.
בחירת דגם
באיזה מודל כדאי להשתמש כדי ליצור תמונות? זה תלוי בתרחיש לדוגמה.
Gemini 2.0 מתאים במיוחד ליצירת תמונות רלוונטיות לפי הקשר, למיזוג של טקסט ותמונות, לשילוב של ידע על העולם ולשימוש בלוגיקה לגבי תמונות. אפשר להשתמש בו כדי ליצור רכיבים חזותיים מדויקים ורלוונטיים להקשר, שמוטמעים ברצפי טקסט ארוכים. אפשר גם לערוך תמונות בצורה שיחה, באמצעות שפה טבעית, תוך שמירה על ההקשר לאורך השיחה.
אם איכות התמונה היא העדיפות העליונה שלכם, Imagen 3 הוא הבחירה הטובה ביותר. Imagen 3 מצטיין ביצירת תמונות ריאליסטיות, פרטים אמנותיים וסגנונות אמנותיים ספציפיים כמו אימפרסיוניזם או אנימה. Imagen 3 הוא גם בחירה טובה למשימות מיוחדות של עריכת תמונות, כמו עדכון הרקעים של מוצרים, התאמת התמונות לרזולוציה גבוהה יותר והוספת מיתוג וסגנון לרכיבים חזותיים. אתם יכולים להשתמש ב-Imagen 3 כדי ליצור סמלי לוגו או עיצובים אחרים של מוצרים ממותגים.
יצירת תמונות באמצעות Imagen 3
Gemini API מספק גישה ל-Imagen 3, המודל האיכותי ביותר של Google ליצירת תמונות לפי טקסט, שכולל מספר יכולות חדשות ומשופרות. אפשר לבצע את הפעולות הבאות באמצעות Imagen 3:
- יצירת תמונות עם פרטים טובים יותר, תאורה עשירה יותר ופחות 'פגמים' מפריעים בהשוואה למודלים קודמים
- הסבר על הנחיות שנכתבות בשפה טבעית
- יצירת תמונות במגוון רחב של פורמטים וסגנונות
- רינדור טקסט בצורה יעילה יותר מאשר במודלים קודמים
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
client = genai.Client(api_key='GEMINI_API_KEY')
response = client.models.generate_images(
model='imagen-3.0-generate-002',
prompt='Fuzzy bunnies in my kitchen',
config=types.GenerateImagesConfig(
number_of_images= 4,
)
)
for generated_image in response.generated_images:
image = Image.open(BytesIO(generated_image.image.image_bytes))
image.show()
curl -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/imagen-3.0-generate-002:predict?key=GEMINI_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"instances": [
{
"prompt": "Fuzzy bunnies in my kitchen"
}
],
"parameters": {
"sampleCount": 4
}
}'
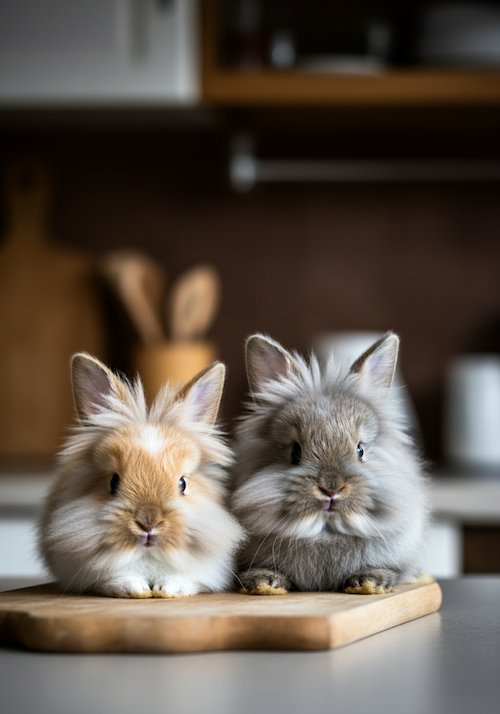
בשלב הזה, Imagen תומך בהנחיות באנגלית בלבד ובפרמטרים הבאים:
הפרמטרים של מודל Imagen
number_of_images
: מספר התמונות שייווצרו, מ-1 עד 4 (כולל). ערך ברירת המחדל הוא 4.aspect_ratio
: שינוי יחס הגובה-רוחב של התמונה שנוצרת. הערכים הנתמכים הם"1:1"
,"3:4"
,"4:3"
,"9:16"
ו-"16:9"
. ערך ברירת המחדל הוא"1:1"
.person_generation
: מאפשרים לדגם ליצור תמונות של אנשים. יש תמיכה בערכים הבאים:"DONT_ALLOW"
: חסימה של יצירת תמונות של אנשים."ALLOW_ADULT"
: יצירת תמונות של מבוגרים, אבל לא של ילדים. זוהי ברירת המחדל.
המאמרים הבאים
- מידע נוסף על כתיבת הנחיות ל-Imagen זמין במדריך להנחיות ל-Imagen.
- למידע נוסף על מודלים של Gemini 2.0, אפשר לעיין במאמרים מודלים של Gemini ומודלים ניסיוניים.