Gemini API는 Gemini 2.0 Flash 실험 버전을 사용한 이미지 생성과 Imagen 3을 사용한 이미지 생성을 지원합니다. 이 가이드를 통해 두 모델을 모두 시작할 수 있습니다.
Gemini를 사용하여 이미지 생성
Gemini 2.0 Flash Experimental은 텍스트와 인라인 이미지를 출력하는 기능을 지원합니다. 이를 통해 Gemini를 사용하여 대화식으로 이미지를 수정하거나 텍스트가 짜여진 출력을 생성할 수 있습니다 (예: 한 번에 텍스트와 이미지가 포함된 블로그 게시물 생성). 생성된 모든 이미지에는 SynthID 워터마크가 포함되며 Google AI 스튜디오의 이미지에는 눈에 보이는 워터마크도 포함됩니다.
다음 예는 Gemini 2.0을 사용하여 텍스트 및 이미지 출력을 생성하는 방법을 보여줍니다.
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
import base64
client = genai.Client()
contents = ('Hi, can you create a 3d rendered image of a pig '
'with wings and a top hat flying over a happy '
'futuristic scifi city with lots of greenery?')
response = client.models.generate_content(
model="gemini-2.0-flash-exp-image-generation",
contents=contents,
config=types.GenerateContentConfig(
response_modalities=['Text', 'Image']
)
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
image = Image.open(BytesIO((part.inline_data.data)))
image.save('gemini-native-image.png')
image.show()
const { GoogleGenerativeAI } = require("@google/generative-ai");
const fs = require("fs");
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY);
async function generateImage() {
const contents = "Hi, can you create a 3d rendered image of a pig " +
"with wings and a top hat flying over a happy " +
"futuristic scifi city with lots of greenery?";
// Set responseModalities to include "Image" so the model can generate an image
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp-image-generation",
generationConfig: {
responseModalities: ['Text', 'Image']
},
});
try {
const response = await model.generateContent(contents);
for (const part of response.response.candidates[0].content.parts) {
// Based on the part type, either show the text or save the image
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
const imageData = part.inlineData.data;
const buffer = Buffer.from(imageData, 'base64');
fs.writeFileSync('gemini-native-image.png', buffer);
console.log('Image saved as gemini-native-image.png');
}
}
} catch (error) {
console.error("Error generating content:", error);
}
}
generateImage();
curl -s -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash-exp-image-generation:generateContent?key=$GEMINI_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"contents": [{
"parts": [
{"text": "Hi, can you create a 3d rendered image of a pig with wings and a top hat flying over a happy futuristic scifi city with lots of greenery?"}
]
}],
"generationConfig":{"responseModalities":["Text","Image"]}
}' \
| grep -o '"data": "[^"]*"' \
| cut -d'"' -f4 \
| base64 --decode > gemini-native-image.png
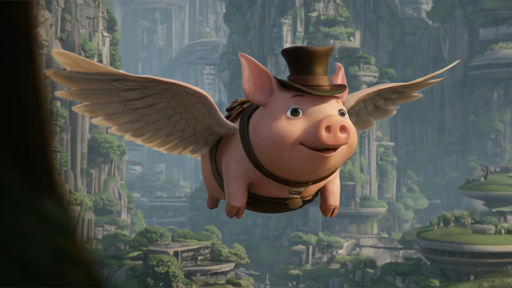
Gemini는 프롬프트와 맥락에 따라 다양한 모드 (텍스트 대 이미지, 텍스트 대 이미지 및 텍스트 등)로 콘텐츠를 생성합니다. 예를 들면 다음과 같습니다.
- 텍스트 이미지 변환
- 프롬프트 예: '배경에 불꽃놀이가 있는 에펠탑 이미지를 생성해 줘'
- 텍스트를 이미지 및 텍스트로 변환(교차 삽입)
- 프롬프트 예: '파에야 레시피를 그림과 함께 생성해 줘.'
- 이미지 및 텍스트에서 이미지 및 텍스트(교차)로 변환
- 프롬프트 예: (가구가 비치된 방의 이미지 포함) "내 공간에 어울리는 다른 색상의 소파가 있나요? 이미지를 업데이트해 주시겠어요?"
- 이미지 수정 (텍스트 및 이미지 대 이미지)
- 프롬프트 예시: '이 이미지를 만화처럼 수정하세요.'
- 프롬프트 예: [고양이 이미지] + [베개 이미지] + '이 베개에 내 고양이 크로스 스티치 만들어 줘.'
- 멀티턴 이미지 수정 (채팅)
- 프롬프트 예: [파란색 자동차 이미지를 업로드하세요.] "이 차를 컨버터블로 바꿔 줘." "이제 색상을 노란색으로 변경하세요."
Gemini를 사용한 이미지 편집
이미지 편집을 실행하려면 이미지를 입력으로 추가합니다. 다음 예는 base64로 인코딩된 이미지를 업로드하는 방법을 보여줍니다. 여러 이미지와 더 큰 페이로드의 경우 이미지 입력 섹션을 확인하세요.
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
import PIL.Image
image = PIL.Image.open('/path/to/image.png')
client = genai.Client()
text_input = ('Hi, This is a picture of me.'
'Can you add a llama next to me?',)
response = client.models.generate_content(
model="gemini-2.0-flash-exp-image-generation",
contents=[text_input, image],
config=types.GenerateContentConfig(
response_modalities=['Text', 'Image']
)
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
image = Image.open(BytesIO(part.inline_data.data))
image.show()
const { GoogleGenerativeAI } = require("@google/generative-ai");
const fs = require("fs");
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY);
async function generateImage() {
// Load the image from the local file system
const imagePath = '/path/to/image.png';
const imageData = fs.readFileSync(imagePath);
const base64Image = imageData.toString('base64');
// Prepare the content parts
const contents = [
{ text: "Hi, This is a picture of me. Can you add a llama next to me?" },
{
inlineData: {
mimeType: 'image/png',
data: base64Image
}
}
];
// Set responseModalities to include "Image" so the model can generate an image
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp-image-generation",
generationConfig: {
responseModalities: ['Text', 'Image']
},
});
try {
const response = await model.generateContent(contents);
for (const part of response.response.candidates[0].content.parts) {
// Based on the part type, either show the text or save the image
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
const imageData = part.inlineData.data;
const buffer = Buffer.from(imageData, 'base64');
fs.writeFileSync('gemini-native-image.png', buffer);
console.log('Image saved as gemini-native-image.png');
}
}
} catch (error) {
console.error("Error generating content:", error);
}
}
generateImage();
IMG_PATH=/path/to/your/image1.jpeg
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
IMG_BASE64=$(base64 "$B64FLAGS" "$IMG_PATH" 2>&1)
curl -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash-exp-image-generation:generateContent?key=$GEMINI_API_KEY" \
-H 'Content-Type: application/json' \
-d "{
\"contents\": [{
\"parts\":[
{\"text\": \"'Hi, This is a picture of me. Can you add a llama next to me\"},
{
\"inline_data\": {
\"mime_type\":\"image/jpeg\",
\"data\": \"$IMG_BASE64\"
}
}
]
}],
\"generationConfig\": {\"responseModalities\": [\"Text\", \"Image\"]}
}" \
| grep -o '"data": "[^"]*"' \
| cut -d'"' -f4 \
| base64 --decode > gemini-edited-image.png
제한사항
- 최상의 성능을 위해 다음 언어를 사용하세요. EN, es-MX, ja-JP, zh-CN, hi-IN
- 이미지 생성은 오디오 또는 동영상 입력을 지원하지 않습니다.
- 이미지 생성이 항상 트리거되지는 않을 수 있습니다.
- 모델은 텍스트만 출력할 수 있습니다. 이미지 출력을 명시적으로 요청해 보세요(예: '이미지 생성', '진행 중 이미지 제공', '이미지 업데이트').
- 모델이 생성을 중단할 수 있습니다. 다시 시도하거나 다른 프롬프트를 사용해 보세요.
- 이미지의 텍스트를 생성할 때는 먼저 텍스트를 생성한 다음 텍스트가 포함된 이미지를 요청하는 것이 가장 좋습니다.
모델 선택
이미지를 생성하려면 어떤 모델을 사용해야 하나요? 사용 사례에 따라 다릅니다.
Gemini 2.0은 문맥과 관련된 이미지를 생성하고, 텍스트와 이미지를 혼합하고, 세계 지식을 통합하고, 이미지에 관해 추론하는 데 가장 적합합니다. 이를 사용하여 긴 텍스트 시퀀스에 삽입된 문맥과 관련성 높은 정확한 시각 자료를 만들 수 있습니다. 대화 중에 컨텍스트를 유지하면서 자연어를 사용하여 이미지를 대화식으로 수정할 수도 있습니다.
이미지 품질이 가장 중요하다면 Imagen 3이 더 나은 선택입니다. Imagen 3은 포토리얼리즘, 예술적 디테일, 인상주의나 애니메이션과 같은 특정 예술적 스타일에 탁월합니다. Imagen 3은 제품 배경 업데이트, 이미지 업스케일링, 시각적 요소에 브랜딩 및 스타일 적용과 같은 특수 이미지 편집 작업에도 적합합니다. Imagen 3을 사용하여 로고나 기타 브랜드 제품 디자인을 만들 수 있습니다.
Imagen 3을 사용하여 이미지 생성
Gemini API는 다양한 새로운 기능이 개선된 Google의 최고 품질 텍스트 이미지 변환 모델인 Imagen 3에 대한 액세스를 제공합니다. Imagen 3은 다음을 실행할 수 있습니다.
- 이전 모델보다 디테일이 더 좋고, 조명이 더 풍부하며, 방해가 되는 아티팩트가 적은 이미지를 생성합니다.
- 자연어로 작성된 프롬프트 이해
- 다양한 형식과 스타일로 이미지 생성
- 이전 모델보다 더 효과적으로 텍스트 렌더링
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
client = genai.Client(api_key='GEMINI_API_KEY')
response = client.models.generate_images(
model='imagen-3.0-generate-002',
prompt='Fuzzy bunnies in my kitchen',
config=types.GenerateImagesConfig(
number_of_images= 4,
)
)
for generated_image in response.generated_images:
image = Image.open(BytesIO(generated_image.image.image_bytes))
image.show()
curl -X POST \
"https://generativelanguage.googleapis.com/v1beta/models/imagen-3.0-generate-002:predict?key=GEMINI_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"instances": [
{
"prompt": "Fuzzy bunnies in my kitchen"
}
],
"parameters": {
"sampleCount": 4
}
}'
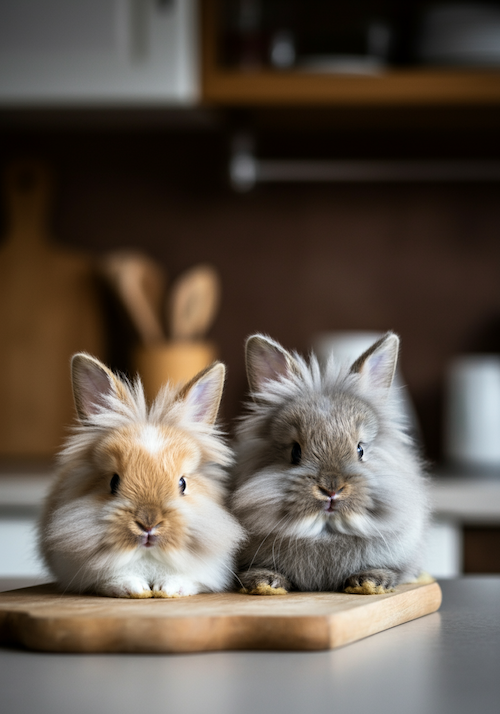
현재 Imagen은 영어로 된 프롬프트와 다음 매개변수만 지원합니다.
Imagen 모델 매개변수
number_of_images
: 생성할 이미지의 수입니다 (1~4, 양 끝값 포함). 기본값은 4입니다.aspect_ratio
: 생성된 이미지의 가로세로 비율을 변경합니다. 지원되는 값은"1:1"
,"3:4"
,"4:3"
,"9:16"
,"16:9"
입니다. 기본값은"1:1"
입니다.person_generation
: 모델이 사람의 이미지를 생성하도록 허용합니다. 다음과 같은 값이 지원됩니다."DONT_ALLOW"
: 사람 이미지 생성을 차단합니다."ALLOW_ADULT"
: 성인의 이미지를 생성하고 어린이의 이미지는 생성하지 않습니다. 이는 기본값입니다.
다음 단계
- Imagen용 프롬프트 작성에 관한 자세한 내용은 Imagen 프롬프트 가이드를 참고하세요.
- Gemini 2.0 모델에 대한 자세한 내용은 Gemini 모델 및 실험용 모델을 참고하세요.